Latest Posts
Greed vs Bravery Based Engineering
It is difficult to find words that accurately describe the cruelty, selfishness and outright evil on display from the White House these days. The guiding principle of Gordon Gekko: is...
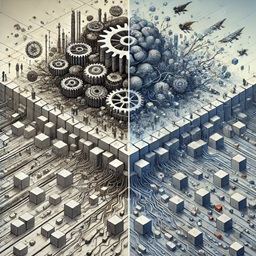
Coupling, Complexity, and Coding
Why is the IT industry obsessed with decoupling? Does breaking systems into smaller parts you can understand individually really make them easier to manage and scale? Today, we the of...
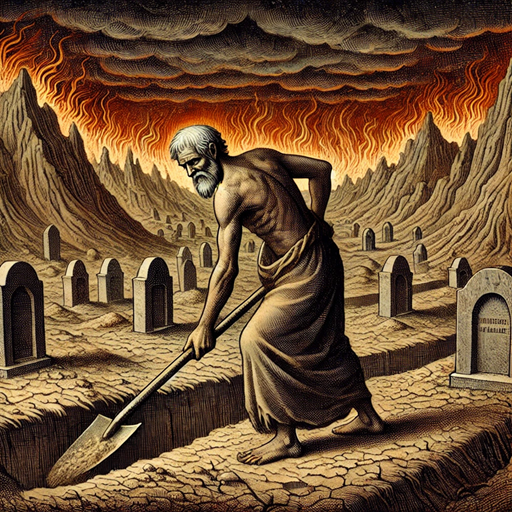
Why are Databases so Hard to Make? Part 4 - Digging up Graves
In my last post about high speed DML, I talked how it is possible to modify tables at the kind of speeds that a modern SSD can deliver. I sketched an outline of an algorithm that can easily us...